I. Selector
Selector는 style 적용의 대상이 될 HTML element를 특정하는 용도로 사용한다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
h1, p { color: red; }
</style>
</head>
<body>
<h1>Hello CSS</h1>
<p>Cascading Style Sheets (CSS) is a style sheet language used for describing the presentation of a document written in a markup language such as HTML or XML.</p>
</body>
</html>

II. Universal Selector
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
* { color: red; }
</style>
</head>
<body>
<h1>Hello CSS</h1>
<p>Cascading Style Sheets (CSS) is a style sheet language used for describing the presentation of a document written in a markup language such as HTML or XML.</p>
</body>
</html>

III. Tag Selector
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
p { color: red; }
</style>
</head>
<body>
<h1>Heading</h1>
<div>
<p>paragraph 1</p>
<p>paragraph 2</p>
</div>
<p>paragraph 3</p>
</body>
</html>
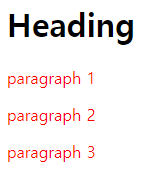
IV. ID Selector
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
#p1 { color: red; }
</style>
</head>
<body>
<h1>Heading</h1>
<div>
<p id="p1">paragraph 1</p>
<p id="p2">paragraph 2</p>
</div>
<p id="p3">paragraph 3</p>
</body>
</html>
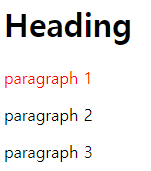
V. Class Selector
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
.abstract { color: red; }
</style>
</head>
<body>
<h1>Heading</h1>
<div class="abstract">
<p>paragraph 1</p>
<p>paragraph 2</p>
</div>
<p>paragraph 3</p>
</body>
</html>
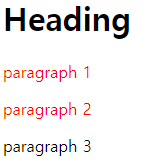
color property가 child element에 상속된다.
HTML element의 class attribute는 여러 개를 지정할 수 있다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
.text-center { text-align: center; }
.text-large { font-size: 200%; }
.text-red { color: red; }
.text-blue { color: blue; }
</style>
</head>
<body>
<h1 class="text-red">Heading</h1>
<p class="text-center text-large text-blue">center large blue</p>
</body>
</html>

위와 같이 class selector로 스타일을 미리 정의하고 HTML element로 원하는 스타일을 가져다 쓰는 방식은 재사용 측면에서 굉장히 유용하다.
V. Attribute Selector
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
a[target] { color: red; }
a[target="_blank"] { color: green; }
</style>
</head>
<body>
<a href="https://www.google.com">구글</a>
<a href="https://www.daum.net" target="_self">다음</a>
<a href="https://www.naver.com" target="_blank">네이버</a>
</body>
</html>

=
이외에도 다양한 방식으로 attribute selector를 설정할 수 있다.
방식 | 설명 |
---|---|
tag[attr~=”value”] | attr 의 값이 “value” 를 공백으로 구분된 단어로 포함하는 element |
tag[attr|=”value”] | attr 의 값이 “value” 와 정확하게 일치하거나 “value-” 를 포함하는 element |
tag[attr*=”value”] | attr 의 값이 “value” 를 포함하는 element |
tag[attr^=”value”] | attr 의 값이 “value” 로 시작하는 element |
tag[attr$=”value”] | attr 의 값이 “value” 로 끝나는 element |
VI. Combinator Selector
combinator는 selector 사이의 관계를 나타내는 기호를 의미한다. 하지만, 어떨 때는 combinator라는 용어 자체가 combinator selector의 줄임말로 쓰여서 특정한 selector를 지칭할 때 사용되기도 한다.
4종류의 combinator가 존재한다.
- descendant combinator (
space
)
- child combinator (
>
)
- adjacent sibling combinator (
+
)
- general sibling combinator (
~
)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
div p {
color: red;
}
div > p {
color: blue;
}
div ~ p {
color: purple;
}
div + p {
color: green;
}
</style>
</head>
<body>
<h3>Structure</h3>
<ul>
<li>div</li>
<ul>
<li>section</li>
<ul>
<li>p (<code>div p</code>)</li>
</ul>
<li>p (<code>div p</code>에도 해당하지만 <code>div > p</code>에 의해 override)</li>
<li>p (<code>div p</code>에도 해당하지만 <code>div > p</code>에 의해 override)</li>
</ul>
<li>p (<code>div ~ p</code>에도 해당하지만 <code>div + p</code>에 의해 override)</li>
<li>p (<code>div ~ p</code>)</li>
<li>p (<code>div ~ p</code>)</li>
</ul>
<br>
<h3>Result</h3>
<div>
<section>
<p>a descendant of div</p>
</section>
<p>a child of div</p>
<p>a child of div</p>
<ul></ul>
</div>
<p>an adjacent sibling of div</p>
<p>an sibling of div</p>
<p>an sibling of div</p>
</body>
</html>
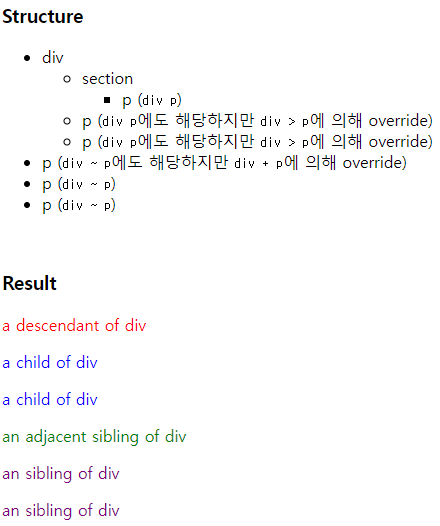
VII. Pseudo-class Selector
pseudo-class는 element의 특정한 상태를 지칭하는 keyword이다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
/* 마우스를 올려놓았을 때 */
a:hover {
font-weight: bold;
}
/* 클릭했을 때 */
p:active {
font-weight: bold;
}
/* 방문한 적 없는 링크 */
a:link {
color:green;
}
/* 방문한 적 있는 링크 */
a:visited {
color:brown;
}
/* focus 상태일 때 */
input[type="text"]:focus {
color:white;
background-color: darkslategray;
}
</style>
</head>
<body>
<a href="http://www.tcpschool.com/css/css_selector_pseudoClass">pseudo-class란?</a><br>
<p>click me</p>
<input type="text" name="zz">
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
input:enabled + span {
color:blue;
}
input:disabled + span {
color: gray;
text-decoration: line-through;
}
input:checked + span {
color: blue;
font-weight: bold;
}
</style>
</head>
<body>
<input type="checkbox" name="" id="" checked><span>Male</span>
<input type="checkbox" name="" id=""><span>Female</span>
<input type="checkbox" name="" id="" disabled><span>Car</span>
</body>
</html>

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
p:nth-child(2n) {
color:green;
}
p:nth-last-child(2) {
color:deeppink;
}
/* p:nth-child(1)과 동일 */
p:first-child {
color:red;
}
/* p:nth-last-child(1)과 동일 */
p:last-child {
color:blue;
}
</style>
</head>
<body>
<p>1st (first)</p>
<p>2nd</p>
<p>3rd</p>
<p>4th</p>
<p>5th</p>
<p>6th (last)</p>
</body>
</html>
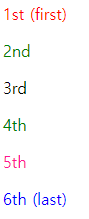
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
input:not([class="required"]) {
color:gray;
}
</style>
</head>
<body>
<h3>필수 항목</h3>
아이디: <input type="text" class="required" value="홍길동">
비밀번호: <input type="password" class="required" value="1111">
<h3>선택 항목</h3>
전화번호: <input type="tel" class="optional" value="010-1234-5678">
</body>
</html>
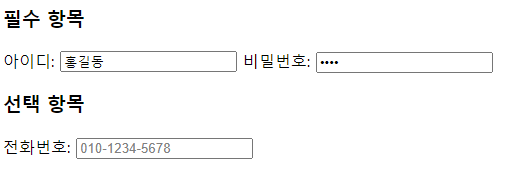
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
input:valid {
font-weight: bold;
}
input:invalid {
background-color: red;
}
</style>
</head>
<body>
전화번호: <input type="tel" value="010-1234-5678" pattern="^\d{3}-\d{4}-\d{4}$"><br>
전화번호: <input type="tel" value="010-1234-567" pattern="^\d{3}-\d{4}-\d{4}$">
</body>
</html>
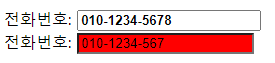
VIII. Pseudo-element Selector
pseudo-element는 element의 특정한 부분을 지칭하는 keyword이다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
p::first-letter {
font-size: 3em;
}
p::first-line {
font-weight: bold;
}
h1::before {
content: "앞";
color: red;
}
h1::after {
content: "뒤";
color: red;
}
p::selection {
background-color: coral;
}
</style>
</head>
<body>
<h1>Title</h1>
<p>Hello, world!<br>Goodbye, world!</p>
</body>
</html>
Reference
[1] https://poiemaweb.com/css3-selector
[2] https://www.w3schools.com/css/css_attribute_selectors.asp
[3] https://www.w3schools.com/css/css_combinators.asp
[4] https://developer.mozilla.org/en-US/docs/Web/CSS/Pseudo-classes
[5] https://developer.mozilla.org/en-US/docs/Web/CSS/Pseudo-elements
Uploaded by N2T
'Frontend > CSS' 카테고리의 다른 글
01. CSS3 기본 문법 (0) | 2022.08.27 |
---|---|
Box Model (1) | 2022.06.05 |
스타일 결정 우선순위 (0) | 2022.06.05 |
Pseudo classess & Pseudo elements (0) | 2022.06.05 |
Combinators (0) | 2022.06.05 |